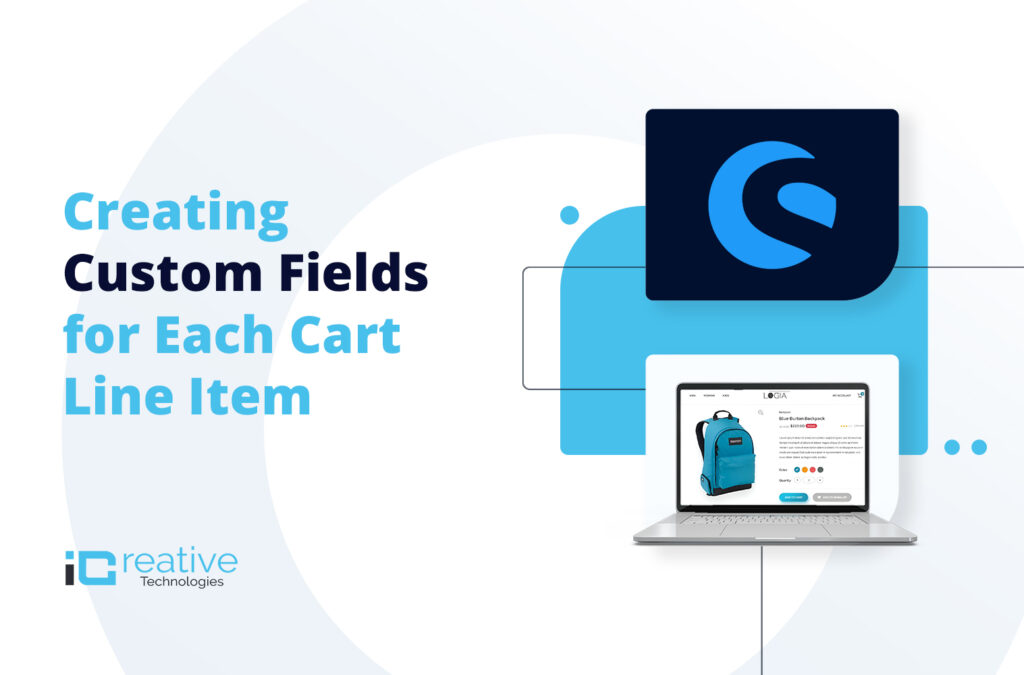
Creating custom fields for each cart line item in Shopware 6 can increase the flexibility and customization of your eCommerce platform, especially when dealing with Shopware checkout processes. Here’s a simple guide on how to do it:
Step 1: Understanding the Requirements
Before diving into the implementation, it’s important to understand what custom fields per cart line item mean. Custom fields let you add extra information to each product in the cart. This can be useful for things like adding special instructions, gift messages, or any other custom data relevant to your business needs.
Step 2: Creating Custom Fields
- For creating the custom fields via code you can refer to this: https://www.icreativetechnologies.com/shopware-6-add-custom-field/
- For creating custom fields via the backend :Â Â
- Access Administration Panel: Log in to your Shopware 6 administration panel.
- Navigate to Custom Fields:
- Go to Settings > System > Custom fields.
- Click on Add Custom Field Set.
- Create a Custom Field Set:
- Name your custom field set (e.g., “Cart Line Item Custom Fields”).
- Assign it to an appropriate entity. For cart line items, you should choose the entity related to line items, such as line_item.
- Add Custom Fields:
- Click on Add Custom Field.
- Define the properties of your custom field:
- Name: A unique identifier for your custom field.
- Type: Choose the appropriate type (e.g., text, number, date).
- Label: A name for the custom field.
- Configuration: Additional settings specific to the type of custom field.
Step 3: Extending the Cart Line Item
To ensure that your custom fields are included in the cart line item, you need to extend the cart functionality in Shopware 6.
- Extend the Cart Service:
- In your plugin, extend the cart service to include your custom fields. This involves modifying the cart data structure to include the new fields.
Example:
<plugin root>/src/Service/CartService.php
namespace YourNamespace\Service;
use Shopware\Core\Checkout\Cart\Cart;
use Shopware\Core\Checkout\Cart\LineItem\LineItem;
class CartService
{
    public function addCustomFieldToCartLineItem(Cart $cart, LineItem $lineItem, array $customFields)
    {
        $lineItem->setPayloadValue(‘customFields’, $customFields);
        $cart->add($lineItem);
    }
}
- Subscribe to Cart Events:
- Listen for cart events to ensure your custom fields are processed correctly..
Example:
<plugin root>/src/Subscriber/CartSubscriber.php
namespace YourNamespace\Subscriber;
use Shopware\Core\Framework\Context;
use Symfony\Component\EventDispatcher\EventSubscriberInterface;
use Shopware\Core\Checkout\Cart\Event\CartAddedEvent;
class CartSubscriber implements EventSubscriberInterface
{
    public static function getSubscribedEvents(): array
    {
        return [
            CartAddedEvent::class => ‘onCartAdded’,
        ];
    }
    public function onCartAdded(CartAddedEvent $event): void
    {
        $cart = $event->getCart();
        $lineItem = $event->getLineItem();
        $context = $event->getContext();
        // Add your custom fields logic here
        $customFields = [‘exampleField’ => ‘exampleValue’];
        $lineItem->setPayloadValue(‘customFields’, $customFields);
    }
}
Step 4: Displaying Custom Fields in the Frontend
To display the custom fields on the front end, you need to modify the template files.
- Override Line Item Template:
- Create a new template file or modify an existing one to include your custom fields.
src/Resources/views/storefront/component/line-item/line-item.html.twigÂ
{% sw_extends ‘@Storefront/storefront/component/line-item/line-item.html.twig’ %}
{% block component_line_item %}
    {{ parent() }}
    {% if lineItem.payload.customFields %}
        <div class=”custom-fields”>
            {% for key, value in lineItem.payload.customFields %}
                <p>{{ key }}: {{ value }}</p>
            {% endfor %}
        </div>
    {% endif %}
{% endblock %}
Conclusion
Setting custom fields for each cart line item in Shopware 6 involves several steps: creating custom fields, extending the cart service, handling cart events, and modifying frontend templates. This guide offers a detailed approach to implementing this feature, enhancing the flexibility and customization of your Shopware 6 store.
Bhavya Shah
Bhavya Shah is a Business Analyst at iCreative Technologies. He specializes in the eCommerce consulting for all business domains. He is working hand-in-hand with developers and clients to produce requirements and specifications that accurately reflect business needs and are technologically achievable.