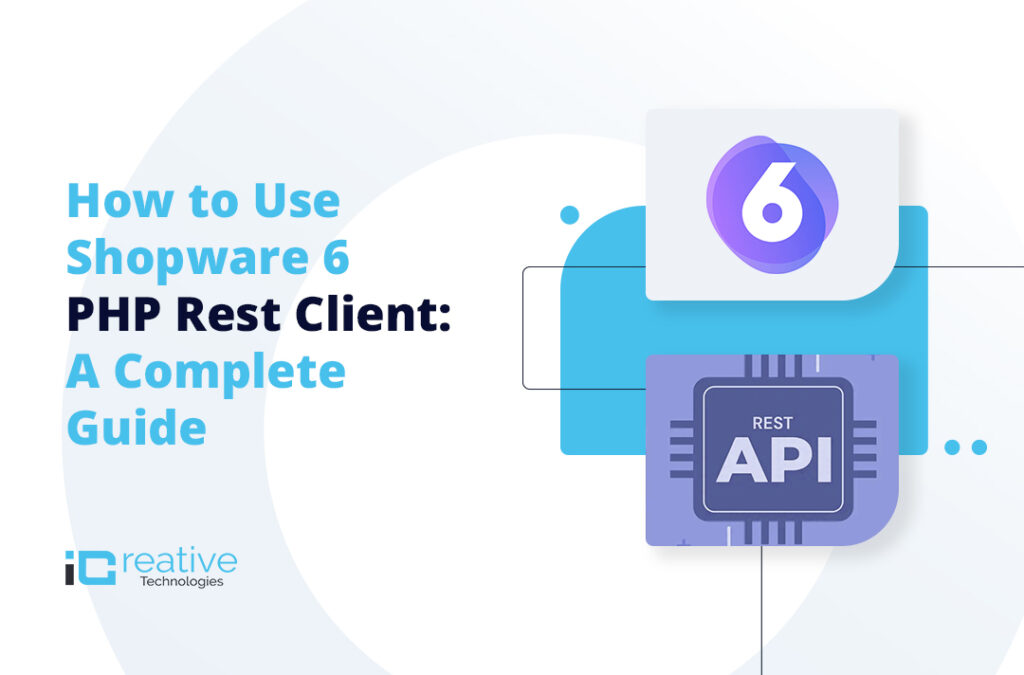
Overview
In this guide, we will learn how to integrate and use a PHP client with Shopware 6 API. This includes using the rest API and creating a client for seamless communication between your Shopware plugin and third-party services.
Prerequisites
To use PHP rest client with the plugin dependency for using Rest client in plugin.
REST API Integration for Shopware 6
Step 1: Plugin Configuration with config.xml
To add a domain URL for a third party into the REST API client via plugin configuration. Define a configuration file to allow users to input the third-party domain URL. In the plugin configuration file, add fields to accept the domain URL.
xml
Copy code
<?xml version=”1.0″ encoding=”UTF-8″?>
<config xmlns:xsi=”http://www.w3.org/2001/XMLSchema-instance”
xsi:noNamespaceSchemaLocation=”https://raw.githubusercontent.com/shopware/shopware/trunk/src/Core/System/SystemConfig/Schema/config.xsd“>
<card>
<title>Basic configuration</title>
<title lang=”de-DE”>Basiseinstellung</title>
<input-field type=”text”>
<name>domainUrl</name>
<defaultValue>your_domain</defaultValue>
<label>Enter domain URl</label>
<label lang=”de-DE”>Domain URl eingeben</label>
</input-field>
</card>
</config>
This setup allows developers to input a domain URL that will be used for API integration.
Step 2: Register the Client in service.xml
Register your ExampleClient into service.xml , inject system config service for get domain url from configuration.
<?xml version=”1.0″ ?>
<container xmlns=”http://symfony.com/schema/dic/services”
xmlns:xsi=”http://www.w3.org/2001/XMLSchema-instance”
xsi:schemaLocation=”http://symfony.com/schema/dic/services http://symfony.com/schema/dic/services/services-1.0.xsd”>
<services>
<service id=”PluginExample\RestApi\ExampleClient” public=”true”>
<argument type=”service” id=”Shopware\Core\System\SystemConfig\SystemConfigService”/>
</service>
</services>
</container>
This step ensures your client is properly integrated and configured to fetch the API URL dynamically.
Step 3: Create the PHP Client
Create the Shopware 6 API client class to handle HTTP requests using the GuzzleHTTP library.
File Path : src/RestApi/
The constructor method initializes the ExampleClient class. It defines a private property $client of type Client and initializes it with a new instance of GuzzleHttp\Client. This client will be used to make HTTP requests.
<?php declare(strict_types=1);
namespace PluginExample\Controller;
use GuzzleHttp\Client;
#[Route(defaults: [‘_routeScope’ => [‘api’]])]
class ExampleClient extends AbstractController
{
public const MM_ENDPOINT = ‘/example/example_create_from_shopware’;
public function __construct(
Private Read
) {
$this->client = new Client();
}
}
Here, the GuzzleHTTP client is initialized to make HTTP requests, an essential step for Shopware 6 API integration.
Step 4: Implement a Method to Call the REST API
Sends an HTTP POST request to a specified endpoint URL.
$this->client->post(…) : Calls the post method on the GuzzleHTTP client ($this->client) to perform an HTTP POST request.
$url . self::MM_ENDPOINT: Constructs the complete URL by appending the endpoint constant self::MM_ENDPOINT to the base URL ($url).
<?php declare(strict_types=1);
namespace PluginExample\Controller;
use GuzzleHttp\Client;
use GuzzleHttp\Exception\GuzzleException;
use Psr\Http\Message\ResponseInterface;
use Shopware\Core\Framework\Context;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\Routing\Annotation\Route;
use Symfony\Component\HttpFoundation\JsonResponse;
use Shopware\Core\System\SystemConfig\SystemConfigService;
#[Route(defaults: [‘_routeScope’ => [‘api’]])]
class ExampleClient extends AbstractController
{
public function __construct(
private readonly SystemConfigService $systemConfigService
) {
$this->client = new Client();
}
public function sendExampleData($payloadData, $context): void
{
$url = $this->systemConfigService->get(‘Plugin.config.domainUrl’);
$this->client->post(
$url . self::MM_ENDPOINT,
[
‘headers’ => [‘Content-Type’ => ‘application/json’],
‘json’ => json_encode($payloadData)
]
);
}
}
This guide explains how to use a PHP client with the Shopware 6 Admin API to interact with third-party services. By using the Shopware 6 API and REST client libraries like GuzzleHTTP, developers can send and receive data effectively.
With this setup, developers can add new features to Shopware and build plugins for different eCommerce needs.
Bhavya Shah
Bhavya Shah is a Business Analyst at iCreative Technologies. He specializes in the eCommerce consulting for all business domains. He is working hand-in-hand with developers and clients to produce requirements and specifications that accurately reflect business needs and are technologically achievable.